Charge a one-off payment with the iframe checkout on your website
Learn the payment flow for a single payment integration where you do not save the customer's card for future use.
1. Create the checkout in the payment gateway
The first step is to perform a POST
request to the payment gateway to create a checkout, with the payment type, amount, currency, and required attributes.
Here is an example request to create a checkout for a single payment.
curl https://eu-test.oppwa.com/v1/checkouts \
-H "Accept: application/json" \
-H "Content-Type: application/x-www-form-urlencoded; charset=UTF-8" \
-d "entityId={channelId}" \
-d "amount=20.00" \
-d "currency=EUR" \
-d "paymentType=DB" \
-d "integrity=true" \
-d "merchantTransactionId=P123" \
-d "billing.street1=Ave. Diagonal 611" \
-d "billing.city=Barcelona" \
-d "billing.country=ES" \
-d "billing.postcode=08028" \
-d "[email protected]" \
-d "customer.ip=2001:8a0:7f4b:1b00:dd4e:2bf6:1fb8:56af" \
-d "customer.givenName=Juan" \
-d "customer.surname=Santos" \
-d "customer.phone=34667666666" \
-d "customer.merchantCustomerId=CUST01" \
-d "threeDSecure.challengeIndicator=04" \
-d "customParameters[CRMCustomerID]=CUST01" \
-d "customParameters[OrderID]=SP-00100" \
-d "customParameters[PaymentType]=SinglePayment" \
-d "customParameters[3DS2_enrolled]=true" \
-d "customParameters[3DS2_flow]=challenge" \
-d "testMode=EXTERNAL" \
-H "Authorization: Bearer {auth_token}"
Replace the example values with your values and replace the {channelId}
and {auth_token}
with your API credentials.
Here are some notes about the parameters in this request
Parameters | Notes |
---|---|
paymentType | Can be DB ("debit"), PA ("preauthorization"), or CD ("credit"). For PA , use the back-office API to capture the payment. |
merchantTransactionId | We recommend that you provide a unique identifier for each transaction. |
billing address | The billing address is required if you use 3DS verification; otherwise, it is recommended. |
customer details | We recommend you supply customer details, including a unique identifier for each customer. |
threeDSecure.challengeIndicator | The recommended value is 04 . This means that 3DS is mandated in your region, and it tells the issuer to define the challenge type. For single purchases, 3DS is not mandatory, but it is recommended. |
customParameters | We recommend adding custom parameters to uniquely identify the customer, order, and purchase type. The custom data you send in these parameters will be returned in the payment response. You can use these parameters to pair and match information from the payment gateway with your business systems. You can create an unlimited number of unique and properly-named custom parameters. |
When you are testing your integration, you can use the following parameters.
Parameters | Notes |
---|---|
customParameters[3DS2_enrolled][3DS2_enrolled] | This parameter is for the test environment only. Set to true for any card to specify that the card is enrolled in 3DS. Or, instead of the 3DS test parameters, you can use 3DS test cards. |
customParameters[3DS2_flow][3DS2_flow] | This parameter is for the test environment only. Set to challenge to force a 3DS challenge, or frictionless . Or, instead of the 3DS test parameters, you can use 3DS test cards. |
testMode | This parameter is for the test environment only. Set to EXTERNAL to send the transaction to the acquirer's test environment. Set to INTERNAL to process the transaction in the gateway only. |
For more details on 3DS testing, see the Gateway 3DS testing guide.
For full details of all the parameters, see Checkout request attributes.
Your successful request will receive a JSON response.
Response Example:
{
"result":{
"code":"000.200.100",
"description":"successfully created checkout"
},
"buildNumber":"d7f3057c29b9a26d5151336767387bb393720d7e@2024-10-14 09:16:49 +0000",
"timestamp":"2024-10-15 14:58:46+0000",
"ndc":"702B930F656317E0D29A22D195F75A59.uat01-vm-tx03",
"id":"702B930F656317E0D29A22D195F75A59.uat01-vm-tx03",
"integrity":"sha384-KnYRC1jbE3C9SMGbJ5eU2Gx+AM9PCaApfqS6lk8MpPlvk9jIii4PFu297dPu4wcy"
}
From this response, you will need the value of the id
and integrity
for the next step.
2. Create the payment form on your web page
To create the payment form on your web page, add the following lines of JavaScript and HTML and enter the variables for your checkout and site.
- This script gets the checkout from the payment gateway. Add the
checkoutId
with the value of theid
and add the value ofintegrity
from the response in Step 1. Replaceanonymous
with the address of your website that loads the COPYandPAY checkout.
<script
src="https://eu-test.oppwa.com/v1/paymentWidgets.js?checkoutId={checkoutId}"
integrity="{integrity}"
crossorigin="anonymous">
</script>
- This HTML displays the checkout. The
shopperResultUrl
is a page on your site where the customer should be redirected after payment processing.
<form action="{shopperResultUrl}" class="paymentWidgets" data-brands="VISA MASTER"></form>
For example, a checkout script could look like this.
<script src="https://eu-test.oppwa.com/v1/paymentWidgets.js?checkoutId=702B930F656317E0D29A22D195F75A59.uat01-vm-tx03"
integrity="sha384-KnYRC1jbE3C9SMGbJ5eU2Gx+AM9PCaApfqS6lk8MpPlvk9jIii4PFu297dPu4wcy"
crossorigin="https://example.com">
</script>
For example, your checkout HTML could look as follows.
<form action="https://myshop.example.com/paid" class="paymentWidgets" data-brands="VISA MASTER"></form>
You can use the same checkout ID multiple times to retrieve a valid payment form. If a customer does
not finish the payment and then reloads the page or uses the browser's back button, you do not need to create a new checkout. However, these actions can generate multiple transactions in the system. For example, there can be one or more failed transactions and one successful transaction from the same checkout ID.
The checkout expires in 30 minutes, and after it expires, you must send a new request to create a checkout and use the new checkoutId
to create the payment form again.
Customise the payment widget
To customise the payment widget to match the look of your own site, see this customisation guide and the advanced options guide.
You can also add extra fields to this form, for example, to obtain the customer details during checkout.
To display some sample customisations, go to demo checkouts. You can obtain the code for the demo checkouts at Demo checkouts on GitHub.
Here is an example of a customised iframe checkout with a payment widget for a single payment.
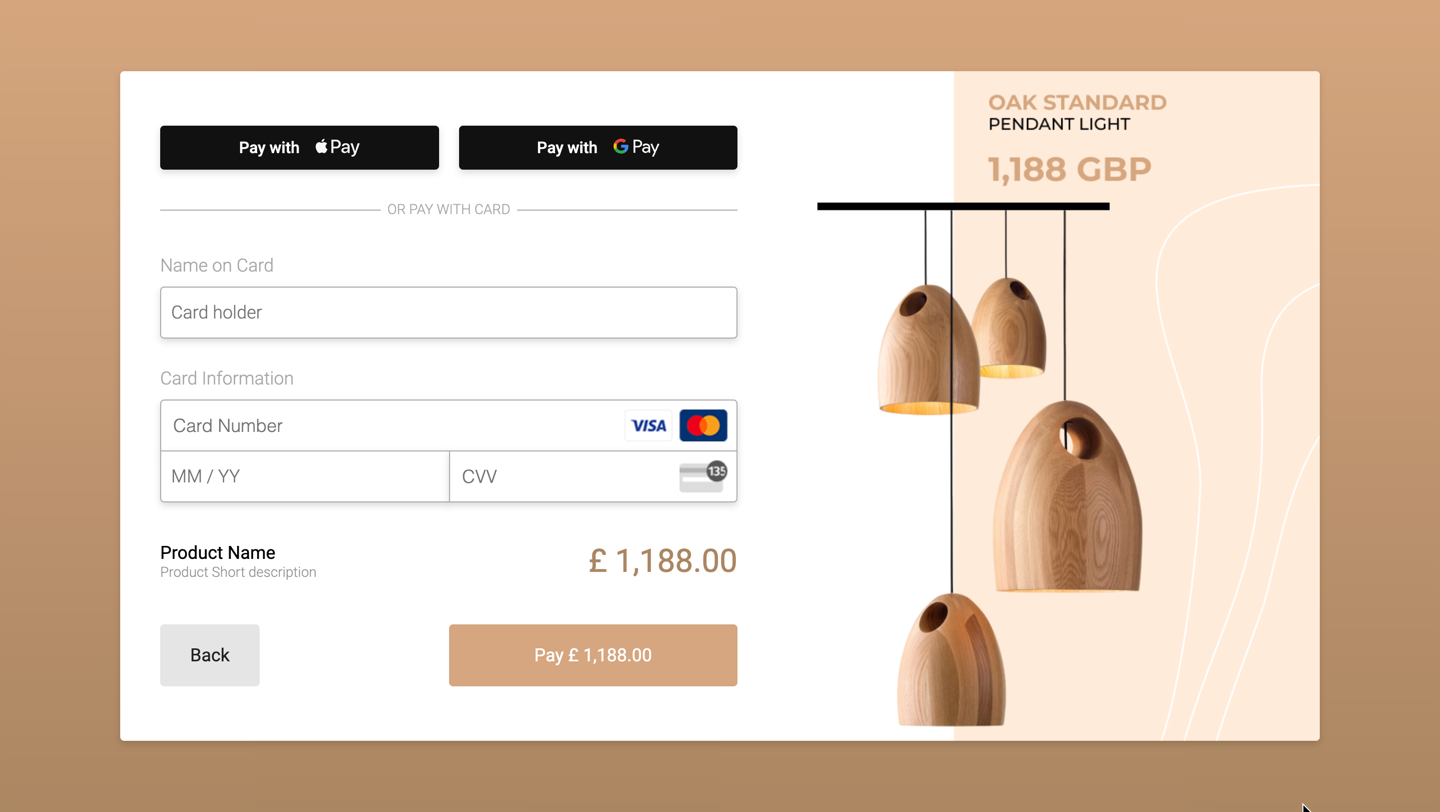
Customised iframe checkout
3. Get the payment status from the checkout in the gateway
When the payment has been processed, CardCorp will redirect the customer to your shopperResultUrl
.
To get the payment status, make a GET request to the payment link of your checkout in the gateway, as follows.
curl -G https://eu-test.oppwa.com/v1/checkouts/{id}/payment \
-d "entityId={channelId}" \
-H "Authorization: Bearer {auth_token}"
Replace the checkout {id}
and the {auth_token}
with your values.
An example of a payment response is as follows.
{
"id":"8ac7a4a1913510db0191363e7df14000",
"processingEntityId":{channelId},
"paymentType":"DB",
"paymentBrand":"MASTER",
"amount":"11.00",
"currency":"EUR",
"descriptor":"4146.4363.5743 ECOMChannel",
"merchantTransactionId":"P123",
"result":{
"cvvResponse":"U",
"code":"000.100.112",
"description":"Request successfully processed in 'Merchant in Connector Test Mode'"
},
"resultDetails":{
"ExtendedDescription":"Approved",
"usedChallengeIndicator":"04",
"clearingInstituteName":"SecureTrading Omnipay Demo",
"ConnectorTxID1":"780744||true|UMCC632791 ||0808|043| ||RECURRING|80||false|false|false|812|",
"connectorId":"422102780744",
"ConnectorTxID3":"422102780744|00|||1||0808150006|||||||||||||Berlin|",
"ConnectorTxID2":"738974||8ac7a0b39131d7470191328119cc0371",
"AcquirerResponse":"00",
"reconciliationId":"4146.4363.5743",
"SchemeResponseCode":"00"
},
"card":{
"bin":"520000",
"binCountry":"MY",
"last4Digits":"0049",
"holder":"John Smith",
"expiryMonth":"02",
"expiryYear":"2027",
"issuer":{
"bank":"PUBLIC BANK BERHAD",
"website":"HTTPS://WWW.PBEBANK.COM/",
"phone":"1-800-22-5555"
},
"type":"CREDIT",
"level":"STANDARD",
"country":"MY",
"maxPanLength":"16",
"binType":"PERSONAL",
"regulatedFlag":"N"
},
"customer":{
"givenName":"John",
"surname":"Smith",
"merchantCustomerId":"CUST11",
"phone":"34667666666",
"email":"[email protected]",
"ip":"2001:8a0:7f4b:1b00:dd4e:2bf6:1fb8:56af"
},
"billing":{
"street1":"Calle Principal 123",
"city":"Barcelona",
"postcode":"08123",
"country":"ES"
},
"threeDSecure":{
"eci":"02",
"version":"2.2.0",
"dsTransactionId":"568de2c8-5077-47fb-9918-8c2ec97518c2",
"challengeMandatedIndicator":"N",
"transactionStatusReason":"17",
"acsTransactionId":"0cae7afe-e50f-445d-a894-855728a4ed9f",
"cardHolderInfo":"",
"authType":"01",
"flow":"challenge",
"authenticationTimestamp":"202408081500",
"authenticationStatus":"Y"
},
"customParameters":{
"3DS_enrolled":"true",
"SHOPPER_EndToEndIdentity":"5cf791d6bc3ee0cb6c43c7cfafa41adfa2f3d12ffc476cc3eddc042c22cbdbef",
"CTPE_DESCRIPTOR_TEMPLATE":"",
"PaymentType":"SinglePayment",
"CRMCustomerID":"CUST11",
"3DS2_flow":"challenge",
"OrderID":"SP-00011"
},
"risk":{
"score":"0"
},
"buildNumber":"174903ec91870d5654938dd40f55da3b35036b23@2024-08-08 12:50:27 +0000",
"timestamp":"2024-08-08 15:00:07+0000",
"ndc":"5D2BC35426C5E617D8D56C5ABEAD6552.uat01-vm-tx04",
"source":"OPPUI",
"paymentMethod":"CC",
"shortId":"4146.4363.5743"
}
To interpret the payment response, see Transaction results.
There is a request limit for payment status calls, after which throttling will occur. You can send two (2) GET
payment requests per minute for each checkout ID.
In the payment response, compare the returned values with the expected values for the following fields: ID(s), amount, currency, brand and type.
After a payment response status check is successful, you cannot use the checkout identifier again. So if you need to get the status, use the Transaction Reports endpoint with the payment id
. You will also need the id
to manage payments with the Backoffice API.
View the full technical integration guide
The gateway integration guide provides a comprehensive playground with code samples in all major programming languages.
Questions? - Visit our website and chat to our payment experts to learn more about integrating our COPYandPAY iFrame checkout.